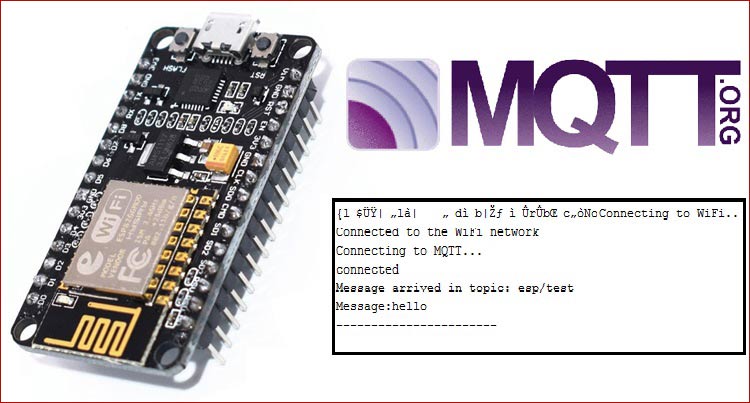
First, you will need to include the MQTT library in your sketch:
#include <PubSubClient.h>
Next, you will need to create a PubSubClient
object and specify the IP address and port of your MQTT broker. You can use the setServer
method to do this:
PubSubClient client;
client.setServer("192.168.1.100", 1883);
Then, you can connect to the MQTT broker using the connect
method of the PubSubClient
object. This method takes the client ID and a boolean value that specifies whether to use clean session mode as arguments. Here is an example:
client.connect("esp8266", true);
Once you are connected to the MQTT broker, you can publish a message to a topic using the publish
method of the PubSubClient
object. This method takes the topic name and the message as arguments. Here is an example:
client.publish("esp8266/topic", "Hello, world!");
You can also subscribe to a topic using the subscribe
method of the PubSubClient
object. This method takes the topic name as an argument. Here is an example:
client.subscribe("esp8266/topic");
When you receive a message on the subscribed topic, the messageReceived
callback function will be called. You can define this function to handle the received message. Here is an example:
void messageReceived(char* topic, byte* payload, unsigned int length) {
// Do something with the received message
}
You can set the messageReceived
callback function using the setCallback
method of the PubSubClient
object. Here is an example:
client.setCallback(messageReceived);
I hope this helps!